728x90
[기본 셋팅]
- UIPractice02Main 생성(UI_ Image)
- guide(UI_Image) 자식으로 생성
- main(빈 오브젝트) 자식으로 생성
- UIScrollview(UI_Image) 자식으로 생성
- content(빈 오브젝트) 부착
- UIGoldCell(UI_Image) 자식으로 생성
UIGoldCell 범위 설정 후 UIScrollview에 Mask 부착하기!
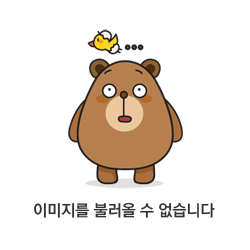
UIGoldCell 만들어주기
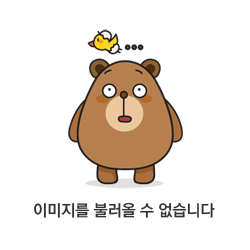
goldbox_data 작성
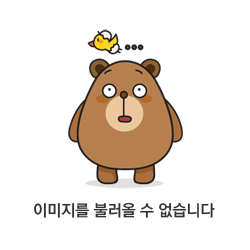
json 파일로도 저장
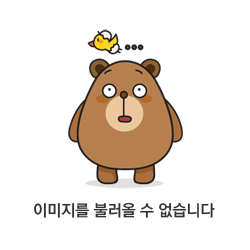
**유의점
AtlasManager에 arrAtlasName 넣을때,
끌어다가 넣지말고(어차피 못 넣음) Element 창에 직접 이름 치기
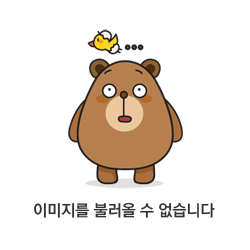
스크립트
Practice02Main
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class UIPractice02Main : MonoBehaviour
{
[SerializeField]
private Practice02UIScrollview scrollview;
private void Start()
{
Practice02DataManager.instance.LoadGoldBoxData();
Practice02AtlasManager.instance.LoadAtlases();
this.scrollview.Init();
}
}
GoldBoxData
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GoldBoxData
{
public int id;
public string name;
public int type;
public int gold_amount;
public string price;
public float price_amount;
public string sprite_name;
}
Practice02DataManager
using Newtonsoft.Json;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using UnityEngine;
public class Practice02DataManager : MonoBehaviour
{
//싱글톤
public static readonly Practice02DataManager instance = new Practice02DataManager();
//GoldBoxData 사전
private Dictionary<int, GoldBoxData> dicGoldBoxDatas = new Dictionary<int, GoldBoxData>();
//goldbox 데이터 불러오기
public void LoadGoldBoxData()
{
//Resources에 저장된 goldbox_data json 파일 (Text형식)
TextAsset asset = Resources.Load<TextAsset>("goldbox_data");
var json = asset.text;
//역직렬화
GoldBoxData[] goldBoxDatas = JsonConvert.DeserializeObject<GoldBoxData[]>(asset.text);
foreach(GoldBoxData goldBoxData in goldBoxDatas)
{
//만들어둔 사전에 goldBoxData의 id를 key로 하여 넣기
this.dicGoldBoxDatas.Add(goldBoxData.id, goldBoxData);
}
Debug.LogFormat("goldbox_data 에셋을 로드했습니다 : {0}",this.dicGoldBoxDatas.Count);
}
//사전에 있는 GoldBoxData List로 가져오기
public List<GoldBoxData> GetGoldboxDataList()
{
return this.dicGoldBoxDatas.Values.ToList();
}
}
UIGoldCell
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using TMPro;
using UnityEngine.U2D;
public class UIGoldCell : MonoBehaviour
{
public enum eGoldType
{
Tiny, Fistful,Pouch, Box,Chest, Vault
}
[SerializeField] private Image icon;
[SerializeField] private TMP_Text txtName;
[SerializeField] private TMP_Text txtPrice;
[SerializeField] private TMP_Text goldAmount;
[SerializeField] private Button btnPrice;
[SerializeField] public eGoldType goldType;
//price가 소수이므로 float으로
public float price;
public int id;
//속성으로 부여하기
public float Price => this.price;
public int Id => this.Id;
public eGoldType GoldType => this.goldType;
//public eGoldType GoldType
//{
// get
// {
// return this.goldType;
// }
//}
public void Init(GoldBoxData data)
{
this.price = data.price_amount;
this.txtName.text = data.name;
this.txtPrice.text = string.Format("US ${0}", this.price);
this.goldType = (eGoldType)data.type;
this.goldAmount.text = data.gold_amount.ToString();
Debug.LogFormat("[UIGoldCell] Init : {0}", this.goldType);
//goldboxData 출력해보기
//Debug.LogFormat("<color=yellow>{1},{2},{3},{4}</color>", data.id, data.name, data.gold_amount, data.price_amount);
//버튼누르면 골드양과 가격 표시
this.btnPrice.onClick.AddListener(() =>
{
Debug.LogFormat("<color=yellow>gold_amout: {0} , price: {1}</color>", this.goldAmount.text, this.txtPrice.text);
});
//icon image에 Atlas sprite 넣기
//AtlasManager에서 Atlas 가져오기
SpriteAtlas atlas = Practice02AtlasManager.instance.GetAtlas("UIGoldBoxAtlas");
//icon에 sprite 넣어주기
this.icon.sprite = atlas.GetSprite(data.sprite_name);
this.icon.SetNativeSize();
}
}
Practice02AtlasManager
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.U2D;
using System;
public class Practice02AtlasManager : MonoBehaviour
{
[SerializeField]
private string[] arrAtlasNames;
//SpriteAtlas : Using UnityEngine.U20; 추가하기
private Dictionary<string, SpriteAtlas> dicAtlases = new Dictionary<string, SpriteAtlas>();
public static Practice02AtlasManager instance;
private void Awake()
{
if(instance != null && instance != this)
{
Destroy(this);
}
else
{
instance = this;
}
DontDestroyOnLoad(this.gameObject);
}
//Atlas 로드하기
public void LoadAtlases()
{
foreach(string atlasName in arrAtlasNames)
{
SpriteAtlas atlas = Resources.Load<SpriteAtlas>(atlasName);
this.dicAtlases.Add(atlasName, atlas);
}
Debug.LogFormat("{0}개의 아틀라스를 로드했습니다.", this.dicAtlases.Count);
}
//SpriteAtlas 가져오기
public SpriteAtlas GetAtlas(string atlasName)
{
return this.dicAtlases[atlasName];
}
}
Practice02UIScrollview
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Practice02UIScrollview : MonoBehaviour
{
[SerializeField]
private GameObject uiGoldboxPrefab;
[SerializeField]
private Transform content;
//UIGoldCell 리스트화
private List<UIGoldCell> cellList = new List<UIGoldCell>();
public void Init()
{
List<GoldBoxData> goldboxDatas = Practice02DataManager.instance.GetGoldboxDataList();
foreach(GoldBoxData data in goldboxDatas)
{
UIGoldCell cell = null;
//content에 자식으로 uiGoldboxPrefab을 가져와 게임오브젝트 생성하기
GameObject go = Instantiate<GameObject>(this.uiGoldboxPrefab, content);
//UIGoldCell 컴포넌트 붙이기
cell = go.GetComponent<UIGoldCell>();
//UIGoldCell에 GoldBoxData 넣어서 초기화
cell.Init(data);
//UIGoldCell 리스트에 추가하기
this.cellList.Add(cell);
}
}
}
스크립트 작성하고 실행한 모습
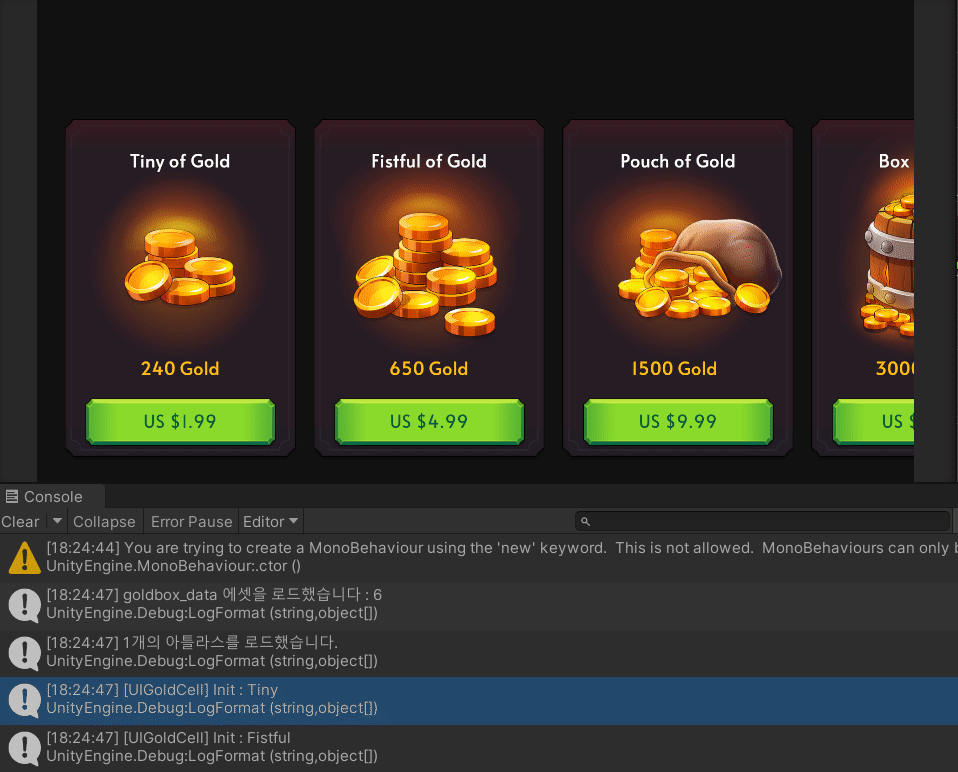
크기나 그런거 때문에 2개의 위치가 이상하다..
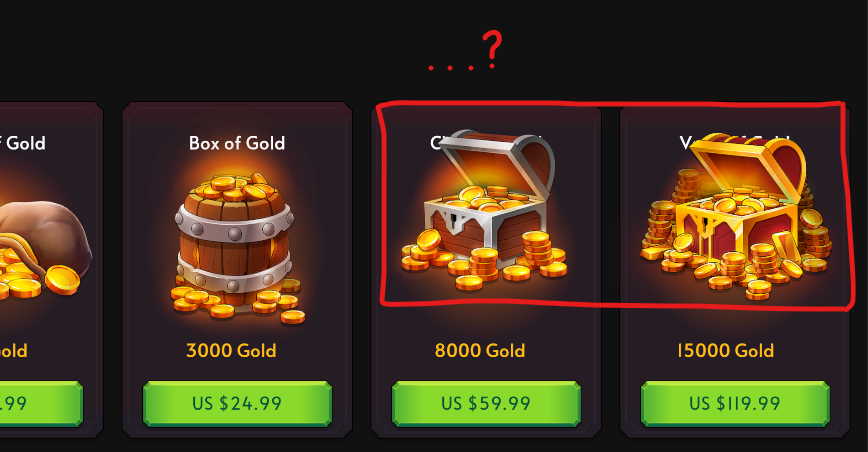
'KDT > 유니티 심화' 카테고리의 다른 글
23/09/11 LearnUGUI (Mission 저장하고 불러오기) (0) | 2023.09.11 |
---|---|
23/09/10 [주말과제] LearnUGUI (ShopGem) (0) | 2023.09.10 |
23/09/07 [복습] LearnUGUI(ShopChest) (0) | 2023.09.08 |
23/09/07 LearnUGUI (구조잡고 정적 스크롤뷰 관리 하는 스크립트 만들기) (0) | 2023.09.07 |
23/09/06 LearnUGUI (Stage 2) (0) | 2023.09.06 |