728x90
ray를 찍은 위치로 바구니 위치 변경(칸에 맞춰서 이동 x)
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using UnityEngine;
public class BasketController : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//마우스 왼쪽 클릭하면(화면 터치) Ray를 만들자
if (Input.GetMouseButtonDown(0))
{
//화면상의 좌표 -> Ray 객체를 생성
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
float maxDistance = 100f;
//Ray를 눈으로 확인
Debug.DrawRay(ray.origin, ray.direction * maxDistance, Color.green, 2f);
//ray와 collider의 충돌 감지
//out매개변수를 사용하려면 변수정의를 먼저 해야함
RaycastHit hit; //충돌된 정보가 담김
//out 키워드를 사용해서 인자로 넣어라
//raycast 메서드에서 연산된 결과를 hit 매개변수에 넣어줌
Physics.Raycast(ray, out hit, maxDistance);
//충돌 되었다면~
if(Physics.Raycast(ray,out hit, maxDistance))
{
Debug.LogFormat("hit point: {0}", hit.point);
this.gameObject.transform.position = hit.point;
this.transform.position = hit.point;
}
}
}
}
ray찍은 위치로 칸의 가운데로 이동(반올림 이용)
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using UnityEngine;
public class BasketController : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//마우스 왼쪽 클릭하면(화면 터치) Ray를 만들자
if (Input.GetMouseButtonDown(0))
{
//화면상의 좌표 -> Ray 객체를 생성
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
float maxDistance = 100f;
//Ray를 눈으로 확인
Debug.DrawRay(ray.origin, ray.direction * maxDistance, Color.green, 2f);
//ray와 collider의 충돌 감지
//out매개변수를 사용하려면 변수정의를 먼저 해야함
RaycastHit hit; //충돌된 정보가 담김
//out 키워드를 사용해서 인자로 넣어라
//raycast 메서드에서 연산된 결과를 hit 매개변수에 넣어줌
Physics.Raycast(ray, out hit, maxDistance);
//충돌 되었다면~
if(Physics.Raycast(ray,out hit, maxDistance))
{
Debug.LogFormat("hit point: {0}", hit.point);
//this.gameObject.transform.position = hit.point;
//바구니의 위치를 충돌한 지점으로 변경
//this.transform.position = hit.point;
//x좌표와 z좌표를 반올림
float x = Mathf.RoundToInt(hit.point.x);
float z = Mathf.RoundToInt(hit.point.z);
//새로운 좌표를 만들고 이동
this.transform.position = new Vector3(x, 0, z);
}
}
}
}
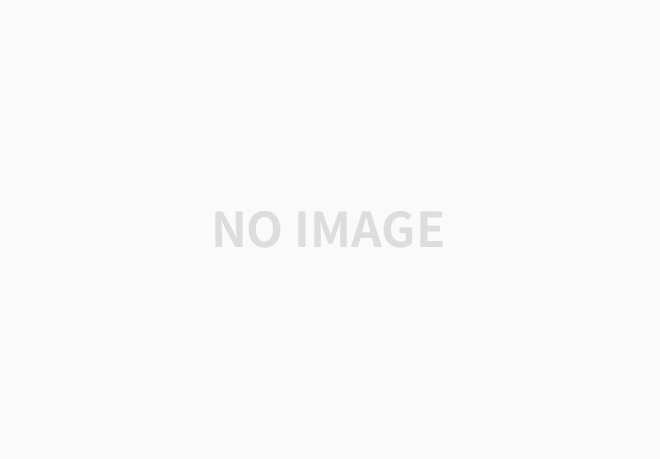
'KDT > 유니티 기초' 카테고리의 다른 글
23/08/08 캐릭터 이동 및 몬스터 공격하기 (0) | 2023.08.08 |
---|---|
23/08/07 과제-복습 (0) | 2023.08.07 |
23/08/06 [주말과제] 캐릭터 위치 이동 및 몬스터 공격(수정) (1) | 2023.08.06 |
23/08/06 유니티 기초 복습(CatEscape, Bamsongi) (0) | 2023.08.06 |
23/08/05 유니티 기초 복습(Roulette, CarSwipe) (0) | 2023.08.05 |